Univalued Binary Tree
Description
A binary tree is univalued if every node in the tree has the same value.
Return true
if and only if the given tree is univalued.
Example 1:
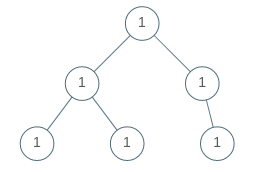
Input: [1,1,1,1,1,null,1] Output: true
Example 2:
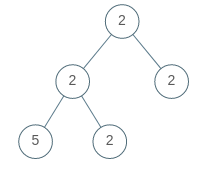
Input: [2,2,2,5,2] Output: false
Note:
- The number of nodes in the given tree will be in the range
[1, 100]
. - Each node's value will be an integer in the range
[0, 99]
.
Solution(javascript)
/**
* Definition for a binary tree node.
* function TreeNode(val) {
* this.val = val;
* this.left = this.right = null;
* }
*/
/**
* @param {TreeNode} root
* @return {boolean}
*/
const isUnivalTree = function (root) {
const aux = (node, prev) => {
if (!node) {
return true
}
if (node.val !== prev) {
return false
}
let isLeftValid = true
let isRightValid = true
if (node.left) {
isLeftValid = aux(node.left, prev)
}
if (isLeftValid && node.right) {
isRightValid = aux(node.right, prev)
}
return isLeftValid && isRightValid
}
if (!root) {
return true
}
return aux(root, root.val)
}